In this tutorial, you will learn how to create a Calendar in Oracle Apex and create events, delete events, etc.
Creating a Table for Calendar
To create a calendar in Oracle Apex, you should have a table containing columns to store event id, event title/description, start date, end date, etc.
Below is an example of the Calendar table:
CREATE TABLE CALENDAR ( EVENT_ID NUMBER PRIMARY KEY, START_DATE DATE, END_DATE DATE, TITLE VARCHAR2(30), CSS_CLASS VARCHAR2(20) ) /
Creating Calendar Region in Oracle Apex
In Oracle Apex, you can create a page for the calendar using the wizard, or you can create a blank page, then create a region and select its type to the Calendar.
Then set the following properties for the Calendar region:
Specify the source type to the table and select the table you created for the calendar.
Click on the Attributes tab/node and specify the start date, end date, and primary key column for the calendar. Below is the screenshot:
Your calendar region creation is complete. Save the changes and run the page to test.
Now you can create the dynamic action on the Calendar region to create events on click.
Creating Events on Date Click
To create events when the user clicks on the calendar dates, you must have two hidden page items to store the start and end dates selected by the user. So create two hidden page items.
Then create a dynamic action on the Date Selected event and create the following True actions:
Create a True action to set the start date to the hidden item.
- Action Type: Set Value
- Set Type: JavaScript Expression
- JavaScript Expression:
this.data.newStartDate
- Affected Items > Selection Type: Items
- Item: P2_START_DATE (hidden item)
- Fire on initialization: Off
Create another True action to Set value:
- Action Type: Set Value
- Set Type: JavaScript Expression
- JavaScript Expression:
this.data.newEndDate
- Affected Items > Selection Type: Items
- Item: P2_END_DATE (hidden item)
- Fire on initialization: Off
Create another True action to execute PL/SQL code to insert the record into the calendar table:
- Action: Execute Server Side Code/ Execute PL/SQL Code
- Add the following code in PL/SQL code section:
begin insert into calendar (id, title, start_date, end_date) values (cal_seq.nextval, 'GENERAL', to_date(:P2_START_DATE, 'YYYYMMDDHH24MISS'), to_date(:P2_END_DATE, 'YYYYMMDDHH24MISS')); end;
Note: You can also insert a value for the CSS_CLASS
column to apply any CSS to the calendar column. For example, to make text bold using CSS, insert the "CSS_BOLD" class and then specify the CSS for this class to make the text bold.
- Items to Submit: P2_START_DATE,P2_END_DATE
- Fire on Initialization: Off
- Action type: Refresh
- Selection Type: Region
- Region: Calendar
- Fire on Initialization: Off
Deleting Calendar Events on Click
- Action Type: Set Value
- Set Type: JavaScript Expression
- JavaScript Expression:
this.data.event.id
- Affected Items > Selection Type: Items
- Item: P2_ID (hidden item)
- Fire on initialization: Off
Create another True action:
- Action Type: Confirm
- Text: Are you sure you want to delete that event?
- Fire on initialization: Off
Create one more True Action:
- Action Type: Execute Server Side code
- PL/SQL Code: add the below code:
begin delete from calendar where id =:P2_ID; end;
- Items to Submit: P2_ID
- Fire on Initialization: Off
- Action type: Refresh
- Selection Type: Region
- Region: Calendar
- Fire on Initialization: Off
Allowing Edit for the Created Events
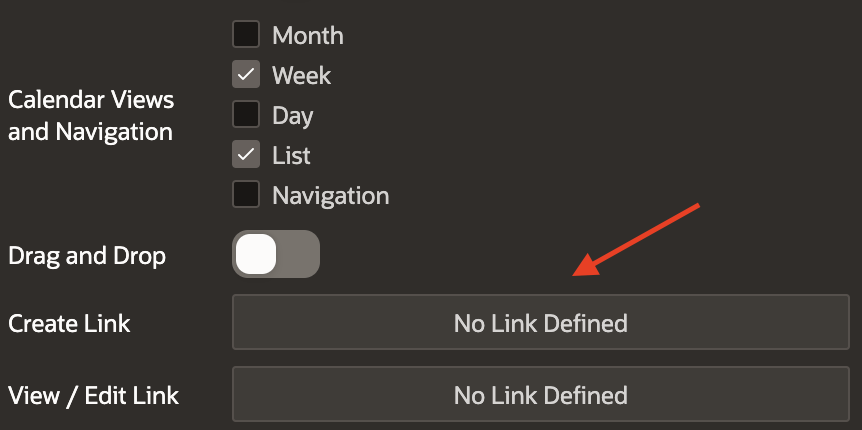
mt
Hello Vinish - thank you for your tutorials!
I'm using Apex 22.1.1 and I have a question about Creating Event on Date Click.
In the beginning, you wrote "Then create a dynamic action on the Date Selected event ...."
I'm not sure what that means. (I usually create dynamic actions from the rendering pane)
I can go to the Dynamic Actions pane, right click to create a new DA, and choose "Date Selected [Calendar] for the When Event. But what is the selection type?
thank you -
mt
mt
never mind; I passed the following in my Create Link and that took care of it for me.
&APEX$NEW_START_DATE.